逆转单链表
逆转单链表,这是一个经典的单链表问题
本节不仅讲如何迭代去逆转单链表
还讲如何使用递归去逆转一部分单链表或者整个单链表
1 | public class ListNode { |
反转链表
迭代实现
1、初始化三个节点prev = null
(作为前置节点)、cur = head
(作为当前节点)、suffix
(作为后置节点)
2、开始循环条件为cur!=null
的循环,cur将一直走到最后一个节点的下一个节点为null的地方
3、先将suffix
指向cur的next,然后将cur
的next指向前一个指针prev
,然后prev
变为cur
,cur
变为suffix
4、返回prev
节点
初始化
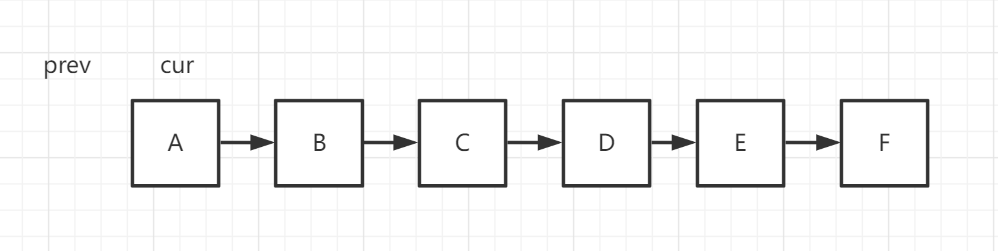
开始循环
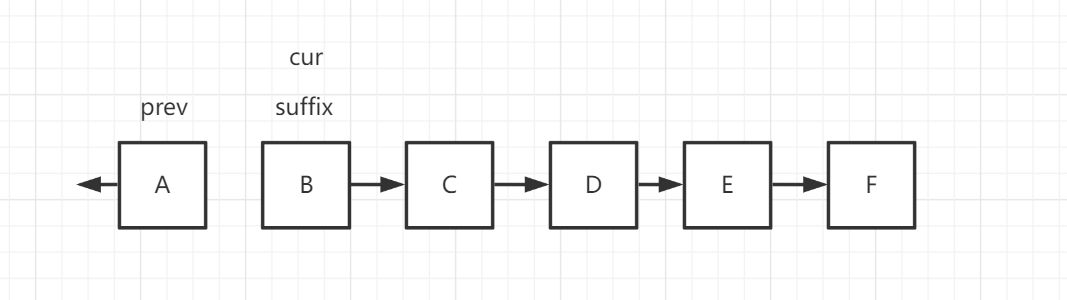
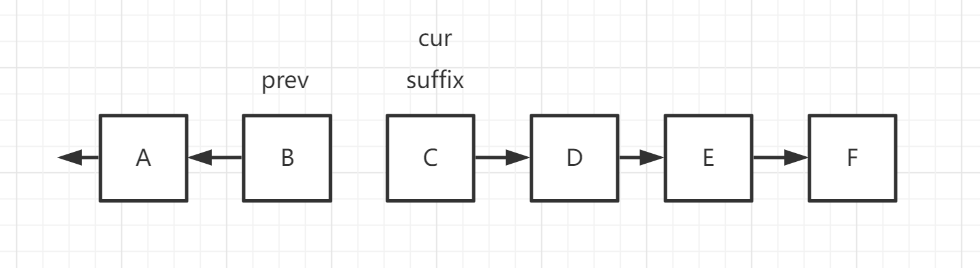
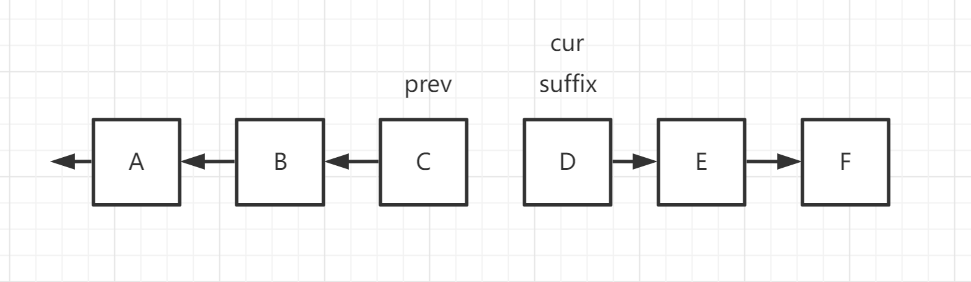
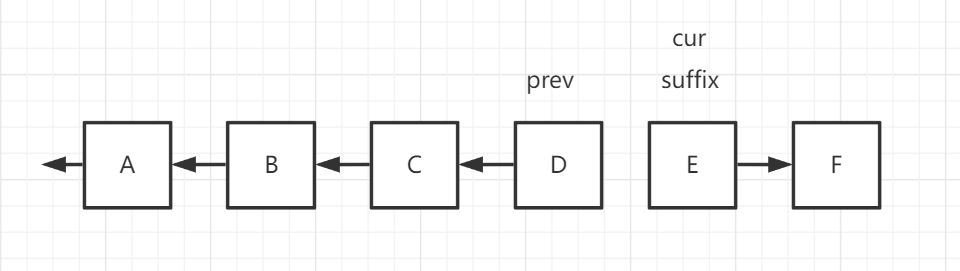
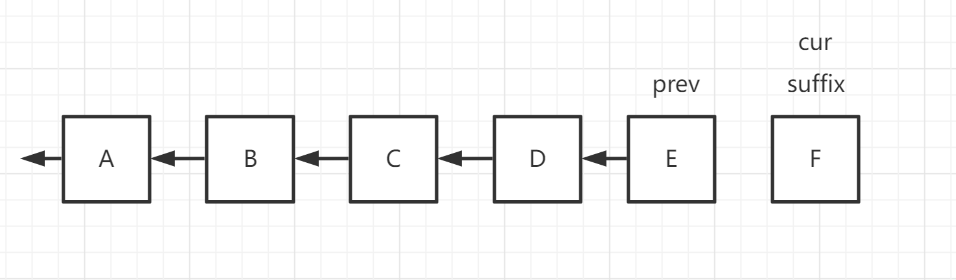
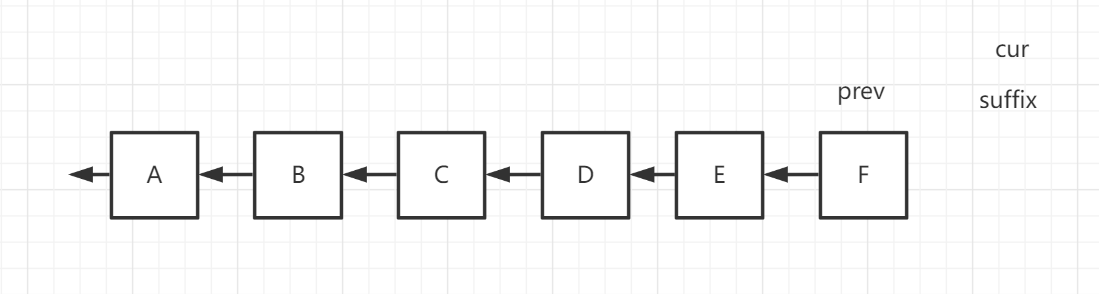
1 | class Solution { |
递归实现
我们的 reverseList
函数定义是这样的:
输入一个节点 head
,将「以 head
为起点」的链表反转,并返回反转之后的头结点。
先递归到源链表的最后一个节点
然后当前的head
节点就是需要加入到反转好的链表中,
head
的next
指针是逆转后的链表的最后一个节点
1 | class Solution { |
反转前n个节点
n<=链表长度
1 | class Solution { |
反转指定索引范围的链表
反转left
到right
部分的链表
注意:这里的 left
是从 1 开始的
如果当left
为1,那就回到上面的那个问题了
所以只需要在reverseBetween
中不断递归直到left
为 1 的时候,right
也就变成了区间长度
1 | class Solution { |