Vue2-基础5
动画效果
在隐藏元素之前执行一段动画
在显示元素之前显示一段动画
1 | <template> |
过渡效果
1 | <template> |
配置代理
发起网络请求我们有以下四种方式
1 | 1.xhr window上的对象,原生 |
这里我们使用axios
来做网络请求
1 | npm i axios |
CORS(Cross-Origin Resource Sharing )
前端中发出ajax请求的时候经常会出现的一种错误,叫做跨域请求
这是因为违背了同源策略
1、同协议
2、主机名
3、端口号
解决方案
1、后端返回数据的时候,加上一些特殊的响应头即可
2、配置代理服务器
服务器之间互相访问是不受同源策略的限制的
所以我们可以配置一个和我们自己的端口号一致的代理服务器
将请求转发给它
让它去请求其他端口上的服务器得到数据后再将其返回
我们可以使用nginx或者借助vue-cli来配置一个代理服务器
这里我们借助vue-cli
开启代理服务器(方式一)
1 | module.exports = { |
- 优点:配置简单,请求资源时直接发给前端(8080)即可。
- 缺点:不能配置多个代理,不能灵活的控制请求是否走代理。
- 工作方式:若按照上述配置代理,当请求了前端不存在的资源时,那么该请求会转发给服务器 (优先匹配前端资源)
开启代理服务器(方式二)
1 | module.exports = { |
- 优点:可以配置多个代理,且可以灵活的控制请求是否走代理。
- 缺点:配置略微繁琐,请求资源时必须加前缀。
插槽
让父组件可以向子组件指定位置插入html结构,也是一种组件间通信的方式,适用于 父组件 ===> 子组件 。
默认插槽
父组件:
1 | <Category> |
子组件:
1 | <template> |
具名插槽
父组件:
1 | <Category> |
子组件:
1 | <template> |
作用域插槽
作用:数据在组件的自身,但根据数据生成的结构需要组件的使用者来决定。
父组件:
1 | <Category> |
子组件:
1 | <template> |
路由
每一个Vue的大型项目都会用到路由,所以这部分十分重要
无路由,无SPA应用
我们回顾一下以前写的web应用的结构
1 | - index.html |
然后每个页面中又有链接可以实现互相跳转,这就是典型的多页面应用
SPA应用
(Single page web application):单页面应用
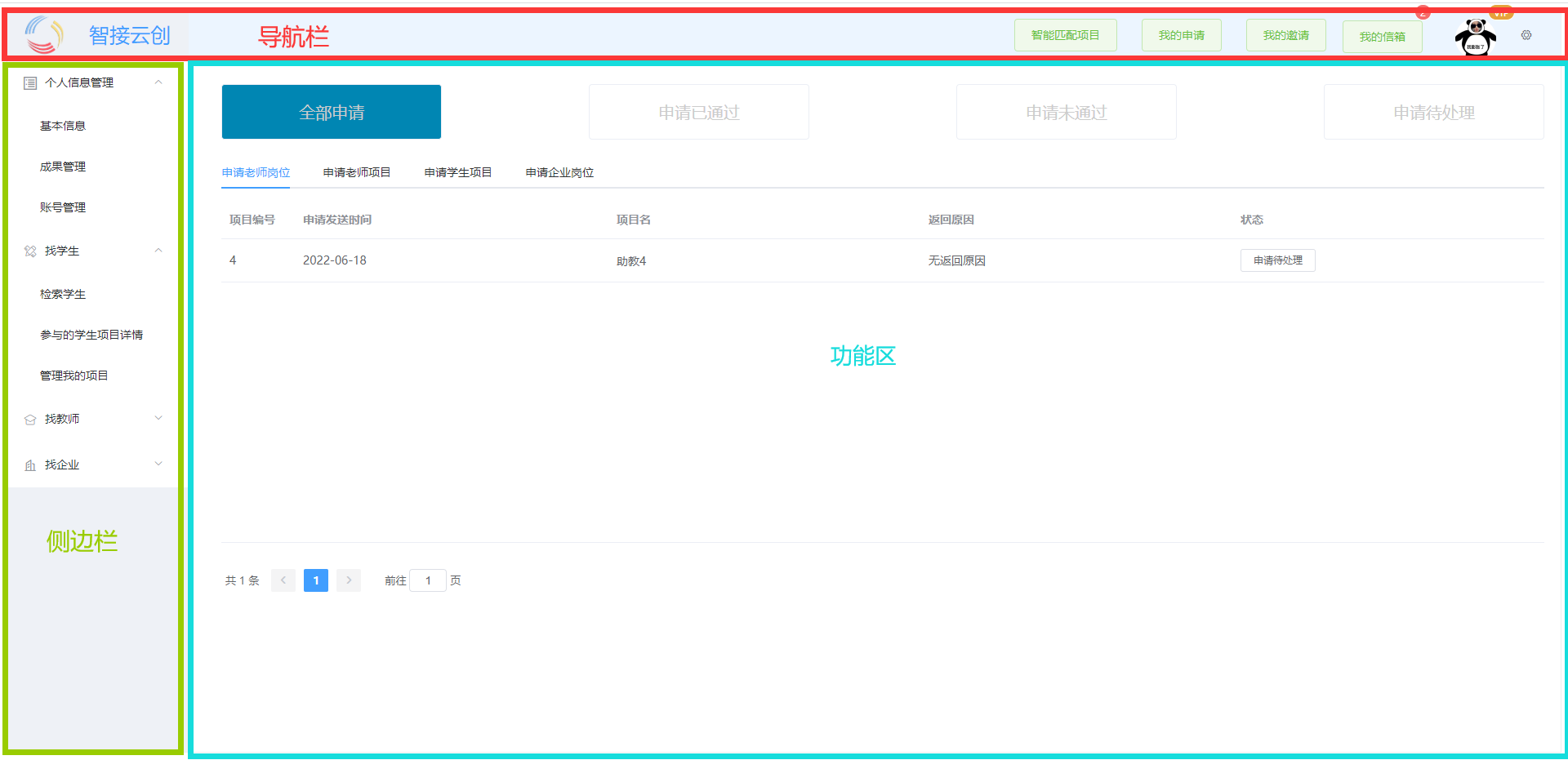
只要点击侧边栏的目录,功能区就会切换到特定的页面,不会刷新页面,实现局部刷新,这就是单页面应用
路由就是用来实现这个切换,路由器就是用来管理这些路由的
概念
路由就是一组key-value的对应关系
多个路由,由一个路由器统一管理
路由分类
后端路由
key就是路径
value就是函数,函数返回对这次请求响应的数据
前端路由
key就是理解
value就是组件,什么样的路径,展示什么样的组件
基本路由
实现以下功能
- 默认显示
pageA
组件 - 点击
pageA
按钮,切换pageA
组件 - 点击
pageB
按钮切换pageB
组件
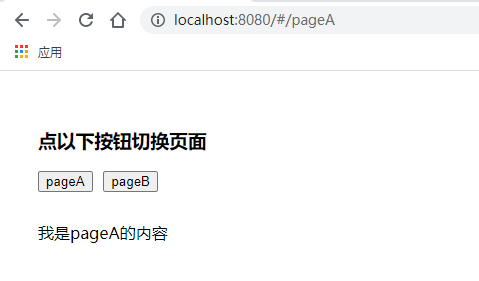
安装Vue-router
1 | npm i vue-router |
安装插件
1 | import Vue from 'vue' |
创建pageA和pageB组件
1 | <template> |
1 | <template> |
创建/router
文件夹并创建index.js
1 | // 该文件专门用于创建整个应用的路由器 |
我们先写声明式路由
1 | <template> |
总结:
1 | 1. 被切换走的组件实际上是被销毁了 |
嵌套路由
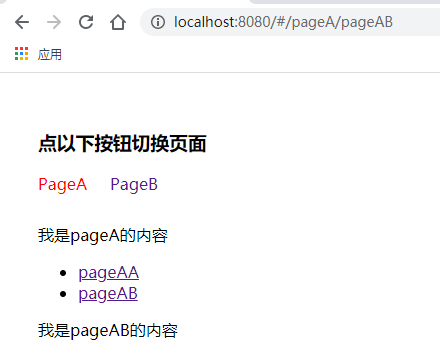
1 | // 该文件专门用于创建整个应用的路由器 |
PageA.vue
1 | <template> |
命名路由
在创建路由器的时候,为路由起名字
1 | // 该文件专门用于创建整个应用的路由器 |
那么在切换的时候,就可以使用名字来选择路由了
1 | <router-link :to="{ |
query参数
1 | <template> |
1 | <template> |
params参数
1 | <li v-for="p in pageList" :key="p.id"> |
1 | // 该文件专门用于创建整个应用的路由器 |
1 | <template> |
query和params的区别
1 | 1. query的对象写法中,path可以是路径也可以是路由名 |
props配置
我们发现读取query
和params
传过来的参数的时候
需要不断使用$route.query.xxx
或者$route.params.xxx
,十分繁琐
如果需要简化,则需要使用props
配置项
1 | { |
replace属性
使用router-link标签实现跳转,浏览器对于历史记录的存储是这样的
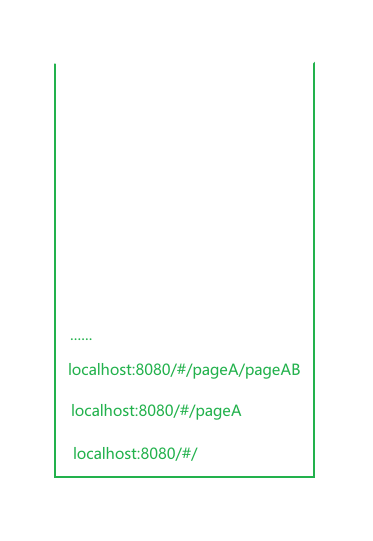
这种模式叫push模式
它将一条条记录压入栈中
如果我们想实现以下这种模式
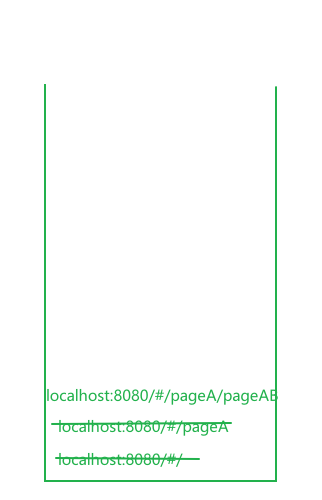
这种模式叫replace模式
它将上一条记录覆盖下一条记录
router-link默认是push模式
增加replace属性以使用replace模式
1 | <router-link class="item" replace active-class="active" to="/pageA">PageA</router-link> |
编程式路由
修改以上案例
1、切换pageA、pageB的a改为button
2、以push模式切换pageA
3、以replace模式切换pageB
4、前进、后退
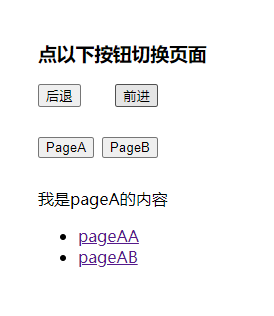
1 | <template> |
编程式路由导航就是不通过router-link实现
而是手动使用router控制的路由跳转
1 | 1. 如果要传入参数,就不能使用字符串了,而是要使用对象写法 |
缓存路由组件
现在再加一个需求在下图出加入一个input组件,然后再pageAA和pageAB之间跳转的时候,input中的数据不会丢失
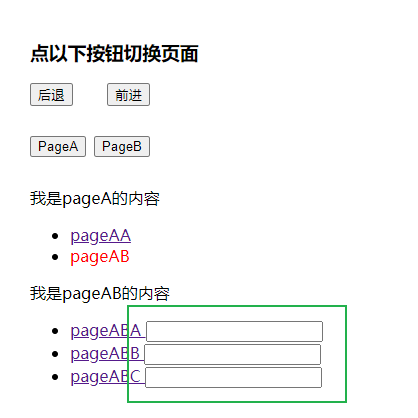
之前我们说过,组件被切换走后,会被销毁
那么我们如何阻止这次的销毁呢
只要在pageA的组件中将router-view用keep-alive包裹即可
1 | <template> |
新的生命周期钩子
在这个地方展示一个透明度一直发生改变的文字
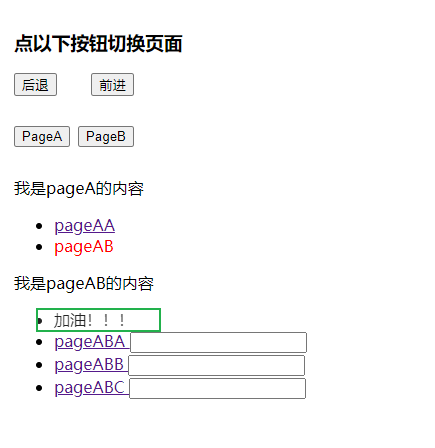
1 | <li :style="{opacity}"> |
然后开启一个定时器,对opacity的值进行操作
1 | mounted() { |
这里其实就出现了问题
我们已经将其设置为了缓存路由
那么当我们切换到其他组件的时候
该定时器还在周而复始的跑,这是不对的
这时候就需要两个新的路由钩子
这是路由独有的两个钩子
1 | activated() { |
路由守卫
全局路由守卫
1 | //全局前置路由守卫————初始化的时候被调用、每次路由切换之前被调用,使用next()放行 |
独享路由守卫
在路由器的路由规则中配置以下逻辑
独享路由守卫没有后置的守卫
1 | { |
组件路由守卫
在组件中加入以下逻辑
1 | //通过路由规则,进入该组件时被调用 |
三个路由守卫可以配合使用哦
history模式和hash模式
我们看到地址栏中始终有#号
#后面的路径都不会发送给服务器
若以后将地址通过第三方手机app分享,若app校验严格,则地址会被标记为不合法。
那么如何切换模式呢?
vue-router默认开启的是hash模式,如果想要切换模式,只需要在创建路由器的时候,传入一个配置项mode: 'history'
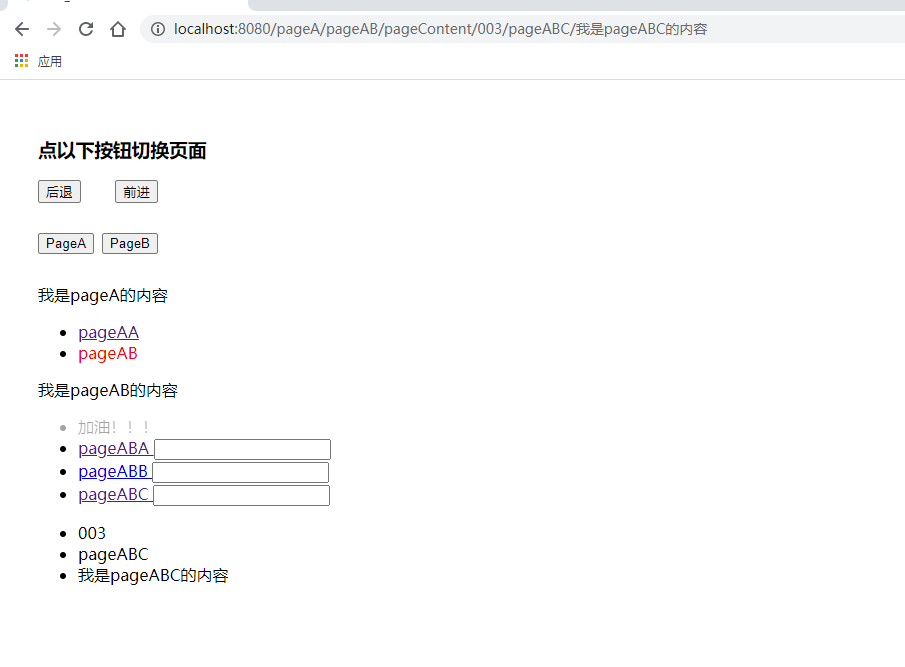
这时候你会发现地址栏的#都不见了
讲到这里,有的小伙伴肯定要说了,那我肯定要使用history模式,这样的路径看着才舒服
history模式下,路径确实舒服,但是hash模式的兼容性比较好,history涉及到一个项目上线的问题
我们使用npm run build
打包
然后你就发现了出现了一个特殊的文件夹
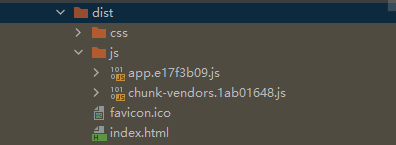
history模式部署上线
我们使用express搭建一个微型的服务器来部署我们的前端项目
1、新建文件夹
mkdir server
2、初始化项目
npm init
3、安装express
npm i express
4、创建static文件夹将dist目录下的所有文件放到其中
5、创建server.js
并编写服务器端代码
1 | const express = require('express'); |
6、启动服务器
node server.js
7、访问localhost:5005
并点击
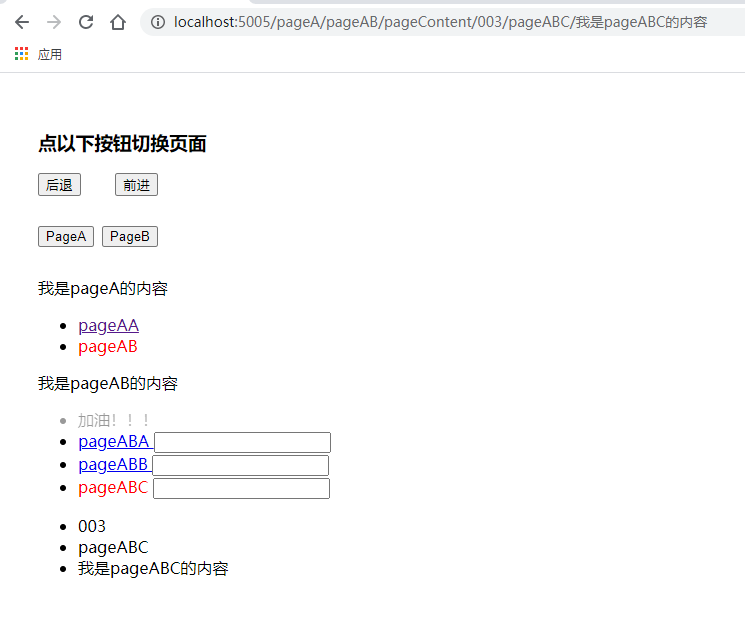
出现问题
这时候这个大问题就出现了,我们刷新页面
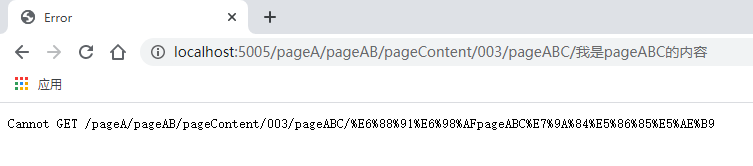
我们发现了404错误,这是为什么呢?因为服务器并没有该资源
hash模式部署上线
那我们使用hash模式再次build,访问尝试
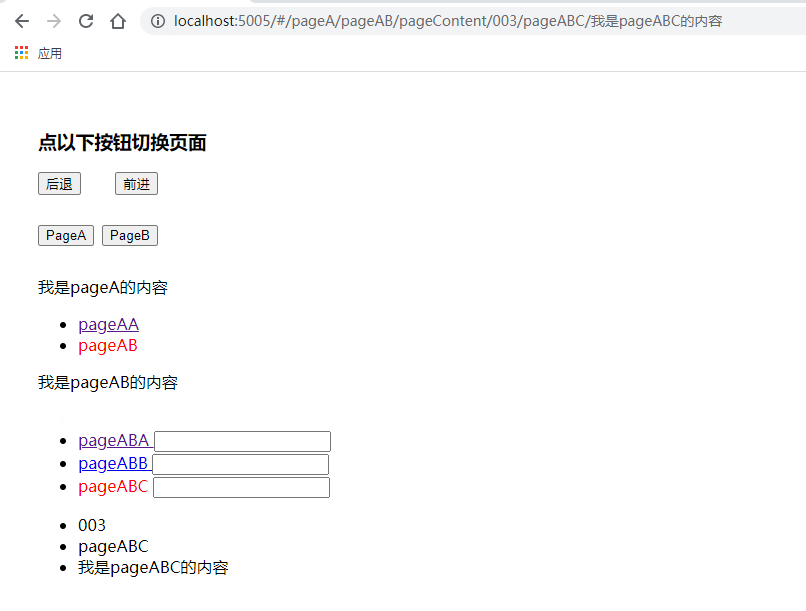
再次刷新,发现并没有出现404
解决history模式的问题
我们再次回到history模式,来解决该问题
这个问题需要后端来配合
我们这里使用nodejs做后端,所以我们使用nodejs的技术来解决问题
安装一个库npm install --save connect-history-api-fallback
1 | const express = require('express'); |
组件库
我们真正开发项目的时候,我们不会所有的页面结构都是自己写,我们会使用第三方的组件库,接下来我来介绍几个比较常用的组件库
- 移动端
- PC端
- Ant Design https://2x.antdv.com/components/overview-cn 蚂蚁金服打造
- ElementUI https://element.eleme.cn/#/zh-CN 饿了么打造
如果想使用这些组件库,我们只需要去这些官网查看官方文档即可