Shiro简介
其实这个就是一个关于做安全管理的框架,他不仅可以和javaEE结合也可以和javaSE结合
提供的功能
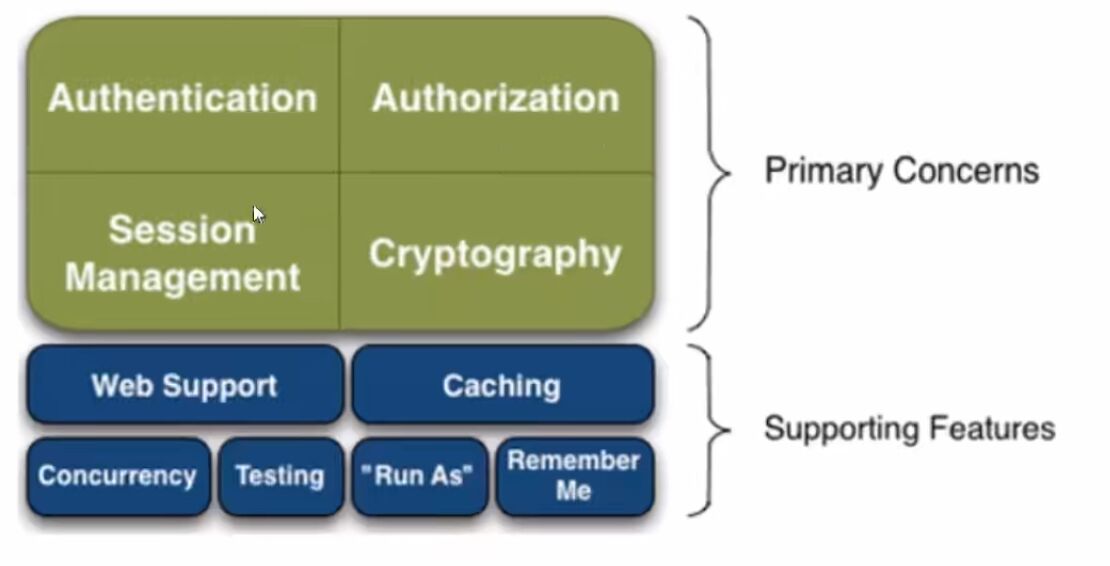
- Authentication:身份认证,登录,验证用户的身份
- Authorization:授权,
- Session Management: Shiro内置的session,对其进行管理
- Cryptography:加密,保证数据的安全性
- Web Support: web支持,可以很好的集成到web环境
- Caching: 缓存,
- Concurrency: 多并发
- Testing:测试
- Remember Me:”记住我”的功能
Shiro结构
我们来观察一下Shiro的结构
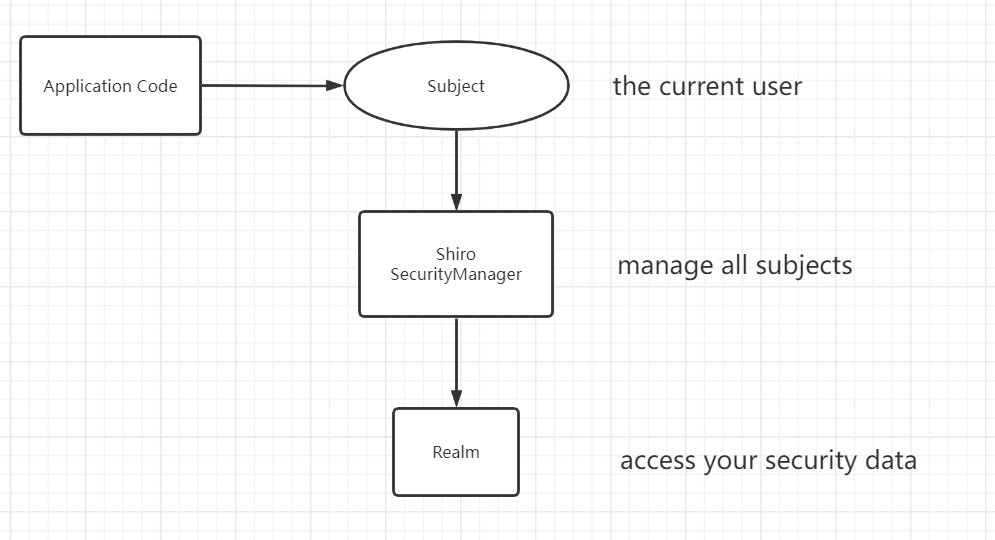
解释一下出现的名词
- subject:与当前应用交互的任何东西都可以是Subject,与Subject的所有交互都会委托给SecurityManager,Subject其实只是一个门面,SecurityManager 才是实际的执行者
- SecurityManager:安全管理器,即所有与安全有关的操作都会与SecurityManager交互,并且它管理着所有的Subject,它是Shiro的核心,它负责与Shiro的其他组件进行交互
- Realm: Shiro从Realm获取安全数据(如用户,角色,权限),就是说SecurityManager 要验证用户身份,那么它需要从Realm 获取相应的用户进行比较,来确定用户的身份是否合法;也需要从Realm得到用户相应的角色、权限,进行验证用户的操作是否能够进行
快速开始(看一下直接过)
导入依赖
1 | <!-- https://mvnrepository.com/artifact/org.apache.shiro/shiro-core --> |
配置文件
log4j.properties
1 | INF0,stdout = |
shiro.ini
1 | [users] |
ShiroQuickStart.java
1 | public class ShiroQuickStart { |
运行输出
1 | 2022-02-17 14:33:37,982 DEBUG [org.apache.shiro.io.ResourceUtils] - |
SpringBoot整合Shiro
先按照以下步骤把基本的环境搭好
- 导入依赖,这里只显示shiro的相关依赖
1 | <dependency> |
- 实体类User.java
1 |
|
- 配置类ShiroConfig.java
1 |
|
- UserRealm.java
1 | public class UserRealm extends AuthorizingRealm { |
- index.html
1 |
|
- user的add页面
1 |
|
- user的del页面
1 |
|
- user的update页面
1 |
|
- MyController.java
1 | package com.lizhi.springbootshiro.contoller; |
- UserMapper.java
user表中就三个字段。id,user_name,password,perms
1 |
|
- UserMapper.xml
1 |
|
登录拦截
登录拦截指的是,未登录的用户不得进入用户的增删改页面
下面是集中拦截规则,一般authc和perms用的比较多
1 | /** |
这是在ShiroFilterFactoryBean中配置的
1 |
|
login.html
1 |
|
MyController中配置路径
1 |
|
用户认证
案例走到这里,你会发现无论怎么登录都是错的,那是因为我们还没有对用户进行认证。
用户认证指的是,当用户登录的时候,对其进行验证
1 |
|
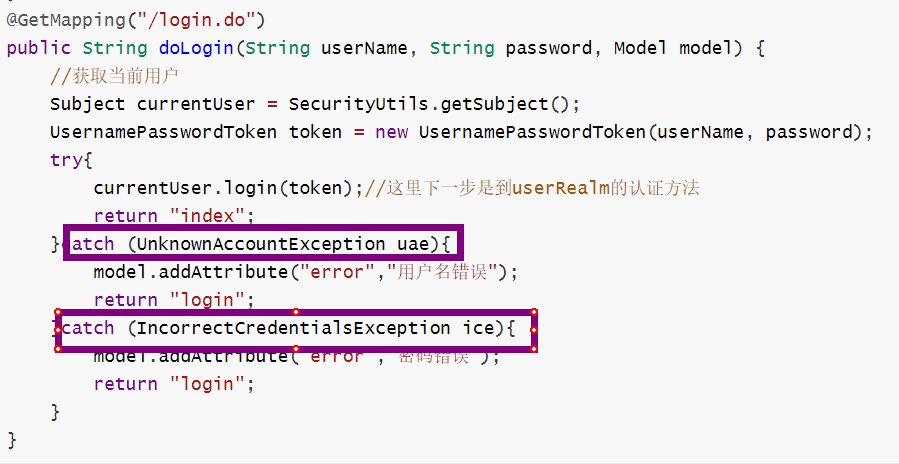
这里插上一嘴,在web环境中,通过subject拿到的session即web框架中的那个session。
但是如果shiro没有使用在web环境的话,内部也是有一个session的,但是那个就不是web中的session了。
用户授权
好啦,现在案例已经可以跑起来了,并且如果账号密码正确的话是可以登录的,并且返回到主页
还记得我们在下图配置的这一串让人一头雾水的配置吗?
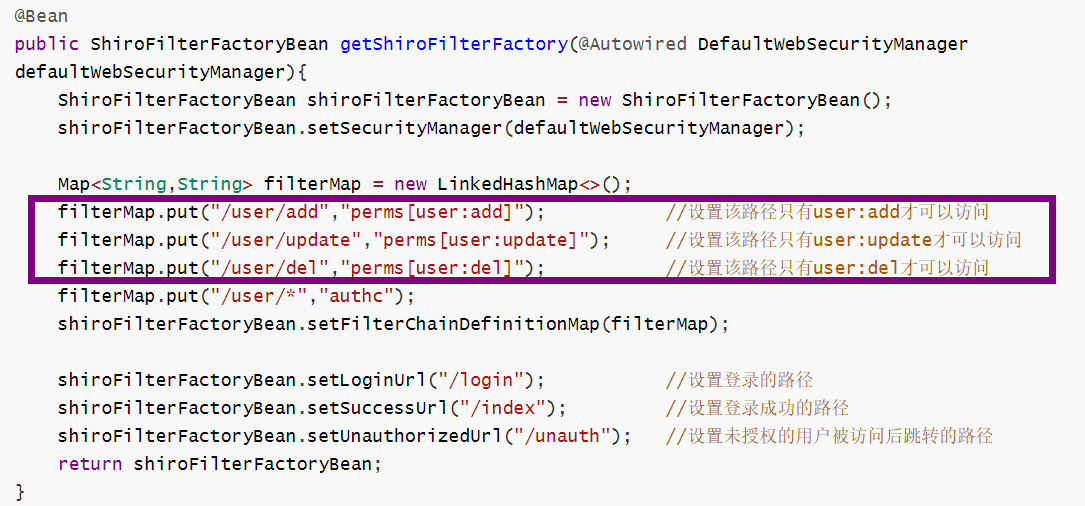
这里就只是配置了一个规则,但是具体登录的用户的授权还没有做,我们现在去完成它吧
1 |
|
现在就完成了所有的配置啦!大家可以尝试一下
这里再多完成一个需求,就是首页只显示用户具有权限的链接
首先需要导入thymeleaf的依赖,然后再导入命名空间xmlns:shiro="http://www.thymeleaf.org/thymeleaf-extras-shiro"
1 | <dependency> |
配置一个bean
1 | //整合thymeleaf |
index.html
1 |
|
注销
大家都应该注意到了,上面的页面中多了一个注销的链接,接下来我们来完成一下注销的功能
1 |
|
是的,你没有看错,就是这么简单,shiro已经将其完美得封装好了
以上